Type Script
December 17, 2023
Exploring TypeScript: A Beginner's Guide
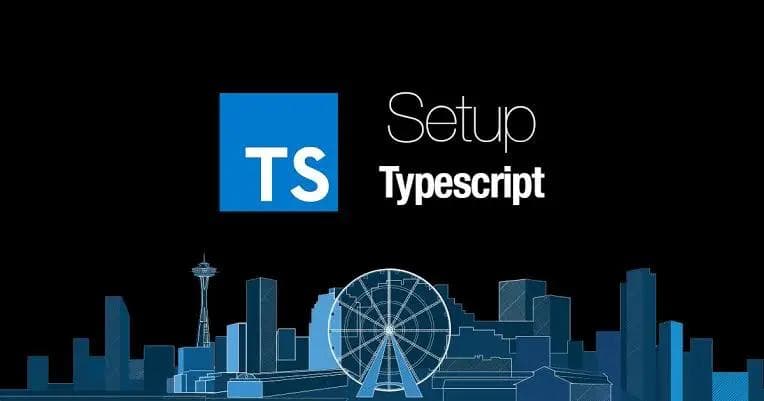
TypeScript is a superset of JavaScript that adds static typing to the language, providing improved tooling and catching potential bugs early in the development process. In this post, we'll go through the basics of TypeScript using a simple example.
Installation
To get started, you need to have Node.js installed on your machine. You can install TypeScript globally using npm:
npm install -g typescript
Your First TypeScript File
Let's create a simple TypeScript file named greet.ts:
// greet.ts
function greet(name: string): string {
return `Hello, ${name}!`;
}
const personName: string = "John";
console.log(greet(personName));
In this example, we've defined a greet function that takes a name parameter of type string and returns a greeting string. We then declare a variable personName of type string and log the result of calling the greet function.
Compiling TypeScript
Next, compile the TypeScript file into JavaScript using the TypeScript compiler (tsc):
tsc greet.ts
This will generate a greet.js file.
Running the JavaScript
Now, you can run the JavaScript file:
node greet.js
You should see the output:
Hello, John!
Understanding TypeScript Features
Static Typing
One of TypeScript's key features is static typing. By specifying types for variables and parameters, we catch errors during development rather than runtime.
Code Organization with Interfaces
Enhance code structure and maintainability using interfaces. Let's modify our example:
interface Person {
name: string;
}
function greet(person: Person): string {
return `Hello, ${person.name}!`;
}
const john: Person = { name: "John" };
console.log(greet(john));
By introducing an interface, we enforce a structure for the Person object, making our code more expressive.
Generics for Reusability
Explore TypeScript generics for creating flexible and reusable functions. Update the greet function to work with various types:
function greet<T>(entity: T): string {
return `Hello, ${entity.toString()}!`;
}
console.log(greet("John"));
console.log(greet(42));
Here, the greet function now accepts a generic type T, allowing it to work with different data types.
Conclusion
Congratulations! You've taken the first steps into the world of TypeScript. This guide provides a foundation for understanding static typing, interfaces, and generics. As you continue your TypeScript journey, explore advanced features and apply them to real-world projects.
Stay curious, and keep coding with TypeScript!