Unity
C#
December 20, 2023
Exploring Unity C#: Compressive Guide with Code Examples
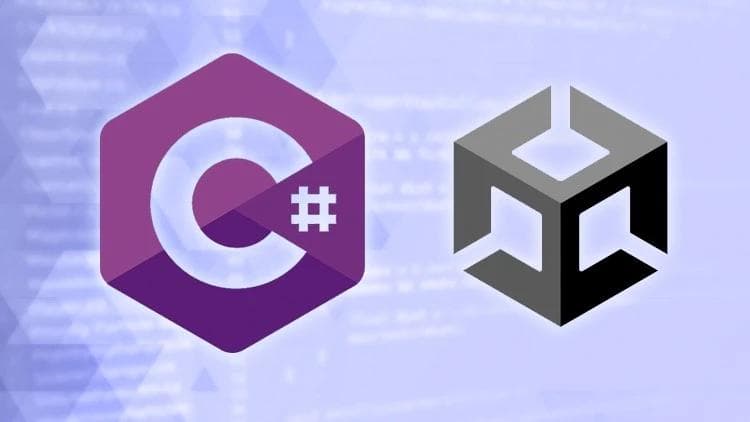
Introduction: Unity, a powerful game development engine, has gained immense popularity for its flexibility and ease of use. One of the key languages used within Unity is C#, a versatile and robust programming language. In this comprehensive blog post, we will delve into the world of Unity C#, exploring its fundamentals and providing practical examples to help you grasp the concepts.
Table of Contents:
Setting Up Your Unity Project:
Before we dive into the code, let's ensure our development environment is set up correctly. Open Unity Hub, create a new project, and choose a template that suits your needs.
Understanding the Unity C# Scripting Basics:
Let's start with the basics of Unity C# scripting. Create a new C# script in your Unity project and open it in your preferred code editor (Visual Studio, Visual Studio Code, etc.). Discuss the structure of a C# script, including namespaces, class declaration, and the essential Start() and Update() methods.
// Example 1: Basic Unity C# Script Structure
using UnityEngine;
public class MyFirstScript : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
Debug.Log("Hello, Unity!");
}
// Update is called once per frame
void Update()
{
// Code for continuous updates
}
}
Variables and Data Types in Unity C#:
Explore the various data types in C# and how they are commonly used in Unity scripts. Discuss the difference between value types and reference types and provide examples of declaring and using variables.
// Example 2: Variables and Data Types in Unity C#
int playerScore = 0;
float playerHealth = 100.0f;
string playerName = "John Doe";
Unity's GameObjects and Components:
In Unity, everything is an object. Discuss the concept of GameObjects and Components, explaining how they form the building blocks of a Unity scene. Provide code examples to create and manipulate GameObjects and their components.
// Example 3: Creating and Manipulating GameObjects
GameObject myCube = GameObject.CreatePrimitive(PrimitiveType.Cube);
myCube.transform.position = new Vector3(0, 2, 0);
User Input and Interactivity:
Explore handling user input in Unity using C#. Discuss Input methods, such as Input.GetKey() and Input.GetMouseButtonDown(), and showcase how to make objects interactive in response to user actions.
// Example 4: Handling User Input in Unity
if (Input.GetKey(KeyCode.Space))
{
// Perform an action when the space key is pressed
}
Physics in Unity C#:
Delve into Unity's physics system and demonstrate how to apply forces, detect collisions, and create realistic interactions within your game.
// Example 5: Applying Forces with Unity Physics
Rigidbody rb = GetComponent<Rigidbody>();
rb.AddForce(Vector3.forward * 10.0f, ForceMode.Impulse);
Advanced Topics:
Briefly touch on more advanced topics, such as coroutines, events, and delegates in Unity C#. Provide practical examples to showcase their applications in game development.
// Example 6: Using Coroutines for Asynchronous Tasks
IEnumerator MyCoroutine()
{
yield return new WaitForSeconds(2.0f);
// Code to execute after waiting for 2 seconds
}
Unity C# Scripting Best Practices:
Discuss best practices for writing clean and efficient Unity C# code. Cover topics such as naming conventions, code organization, and the importance of comments. Encourage readers to follow industry-standard practices to enhance code readability and maintainability.
// Example 7: Following Naming Conventions
int playerHealth = 100;
Debugging Techniques in Unity C#:
Explore different debugging tools and techniques available in Unity. Showcase the effective use of Debug.Log(), breakpoints, and Unity's built-in Profiler to identify and fix issues in your scripts.
// Example 8: Debugging with Unity's Debug.Log
Debug.Log("Player position: " + player.transform.position);
Scriptable Objects in Unity:
Introduce Scriptable Objects, a powerful Unity feature that allows for flexible data representation and sharing between different game objects. Provide examples of creating and using Scriptable Objects to store and manage data.
// Example 9: Creating a Scriptable Object
[CreateAssetMenu(fileName = "NewData", menuName = "Custom Data")]
public class CustomData : ScriptableObject
{
public int value;
}
Unity Events and Messaging System:
Explore Unity's event system and messaging to facilitate communication between different components and game objects. Show how to define and invoke custom events to decouple code and improve modularity.
// Example 10: Defining and Invoking Unity Events
public class EventExample : MonoBehaviour
{
public UnityEvent onCustomEvent;
void Start()
{
// Invoke the custom event
onCustomEvent.Invoke();
}
}
Optimizing Performance in Unity C#:
Discuss performance optimization techniques to ensure smooth gameplay. Cover topics like object pooling, avoiding unnecessary calculations, and using Unity's Job System for parallel processing.
// Example 11: Object Pooling for Efficient Memory Usage
List<GameObject> objectPool = new List<GameObject>();
void SpawnObject()
{
GameObject obj = GetObjectFromPool();
// Perform object initialization and activation
}
Networking in Unity C#:
Briefly introduce networking concepts in Unity, including multiplayer game development. Discuss the use of Unity's Networking API or third-party libraries for creating online multiplayer experiences.
// Example 12: Networking in Unity
void StartServer()
{
// Code to start a multiplayer server
}
void ConnectToServer()
{
// Code to connect to a multiplayer server
}
Continuous Learning and Community Involvement:
Emphasize the importance of staying updated with the latest Unity features, C# improvements, and industry trends. Encourage readers to participate in Unity forums, attend conferences, and engage with the community to enhance their skills.
Conclusion:
Conclude the blog post by summarizing the advanced topics covered and reiterating the significance of continuous learning in the dynamic field of Unity game development. Thank readers for their time and invite them to share their thoughts and questions in the comments.
This extended blog post provides a more in-depth exploration of Unity C#, offering readers a comprehensive guide to both fundamental and advanced concepts.
Happy coding and game development!