Next Js
December 17, 2023
Next.js: The Framework for Building Blazing-Fast Websites and Apps
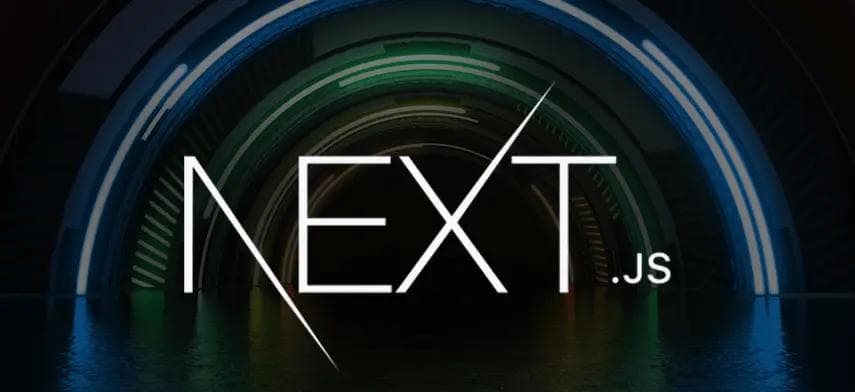
Next.js is a React framework that takes the power and flexibility of React to a whole new level. It provides a plethora of features and functionalities that make building modern web applications a breeze, from server-side rendering and static site generation to data fetching and routing. In this blog post, we'll delve into the world of Next.js, exploring its key features and capabilities with code examples to illustrate their practical applications.
1. Server-Side Rendering (SSR) and Static Site Generation (SSG):
Next.js offers two powerful rendering options: SSR and SSG. SSR pre-renders pages on the server for each request, delivering lightning-fast initial page loads and optimal SEO. SSG pre-renders pages at build time, resulting in even faster initial loads and reduced server load. You can choose the right approach based on your specific needs, whether it's dynamic content or optimal performance.
Example:
// pages/index.jsx
export async function getStaticProps() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return { props: { data } };
}
export default function Home({ data }) {
return (
<div>
<h1>Welcome to Next.js!</h1>
<ul>
{data.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
}
This code snippet demonstrates SSG in action. The getStaticProps function fetches data from an API at build time and passes it as props to the Home component. This ensures the page loads instantly with the data readily available.
2. Data Fetching:
Next.js makes data fetching a breeze with built-in support for various methods like getStaticProps (for SSG), getServerSideProps (for SSR), and useSWR (for client-side fetching). You can seamlessly fetch data from APIs, databases, or any other source and use it to populate your components.
Example:
// pages/posts/[id].jsx
export async function getServerSideProps({ params }) {
const { id } = params;
const response = await fetch(`https://api.example.com/posts/${id}`);
const post = await response.json();
return { props: { post } };
}
export default function Post({ post }) {
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
}
This code snippet illustrates SSR data fetching. The getServerSideProps function fetches the post data based on the id parameter and passes it to the Post component. This ensures the specific post content is rendered on the server for each request.
3. Routing:
Next.js provides a powerful routing system that allows you to define custom routes and handle navigation within your application. You can create dynamic routes, catch-all routes, and even handle nested routes with ease.
Example:
// pages/blog/[slug].jsx
export async function getStaticProps({ params }) {
const { slug } = params;
const response = await fetch(`https://api.example.com/blog/${slug}`);
const blogPost = await response.json();
return { props: { blogPost } };
}
export default function BlogPost({ blogPost }) {
return (
<div>
<h1>{blogPost.title}</h1>
<p>{blogPost.content}</p>
</div>
);
}
This code snippet shows a dynamic route for blog posts. The [slug] placeholder captures the post slug from the URL and uses it to fetch the relevant post data. This allows you to create individual pages for each blog post with dynamic content.
4. Additional Features:
Next.js is packed with additional features that make it a developer-friendly and versatile framework. These include:
Automatic code splitting: Improves performance by loading only the code needed for each page.
Image optimization: Reduces image file sizes for faster loading times.
Headless CMS integration: Integrates seamlessly with popular headless CMS platforms like Contentful and Prismic.
TypeScript support: Enhances code development with type safety and code completion.
5. API Routes and Serverless Functions:
Next.js allows you to create server-side API routes using Node.js functions. These routes handle data requests and responses without needing a separate server backend. This simplifies your architecture and leverages serverless functions for scalability and cost efficiency.
Example:
// api/data.jsx
export default async function handler(req, res) {
const data = await fetch('https://external-api.com/data');
res.json(data);
}
This code defines an API route that fetches data from an external API and returns it as JSON. You can then call this route from your components or other applications.
6. Incremental Static Regeneration (ISR):
ISR takes SSG a step further by re-rendering pages when specific data changes on the server. This ensures your content remains fresh and up-to-date without sacrificing the benefits of static generation.
Example:
// pages/news.jsx
export async function getStaticProps() {
const response = await fetch('https://api.example.com/latest-news');
const news = await response.json();
return { props: { news }, revalidate: 60 }; // Revalidate every 60 seconds
}
export default function News({ news }) {
// ... render news ...
}
This code illustrates ISR for a news page. The getStaticProps function fetches news data and sets a revalidation window of 60 seconds. If the data changes within this window, the page will be automatically re-rendered with the latest updates.
7. Custom Next.js App Architecture:
Next.js provides flexibility to customize your application architecture, including folder structures, data fetching strategies, and component organization. This allows you to tailor your development experience and project setup to your specific needs.
Example:
Create custom folders for components, layouts, and utilities, leveraging pages for routes and separating data fetching logic into dedicated modules. This promotes code organization and maintainability for larger projects.
8. Advanced SEO Enhancements:
Next.js offers built-in tools and features to optimize your application for search engines. These include automatic sitemap generation, dynamic meta tags, and image optimization, resulting in better organic visibility and improved user experience.
9. Continuous Integration and Deployment (CI/CD):
Next.js integrates seamlessly with popular CI/CD platforms like Vercel and Netlify. This allows for automated builds, deployments, and serverless configurations, streamlining your development workflow and ensuring production-ready applications.
Conclusion:
These additional features showcase the true power and flexibility of Next.js. With its wide range of capabilities, Next.js equips developers to build high-performance, scalable, and SEO-friendly web applications tailored to their unique needs. So, why not dive deeper and unlock the full potential of this versatile framework?
Remember, this is just a starting point. As you explore Next.js further, you'll discover a vast ecosystem of libraries, plugins, and community resources that can help you craft exceptional web experiences. Happy coding!